IEnumerable
IEnumerable:可枚举类型,一个方法GetEnumerato(),实现该接口的类就可以对它进行遍历。
IEnumerator:枚举器,一个属性Current,两个方法MoveNext(),Reset()。
扩展方法
在不改变源码的基础上为类添加新的方法
1 | namespace LinqTest |
Linq
- linq to object 查询内存中的数据
- linq to sql 查询数据库中的数据
- linq to xml 查询xml文件
1 | // 原始数据 |
Where 指定条件查询数据
1 | List<Student> students1 = studentList.Where(stu => stu.Age > 18).ToList(); |
ForEach 遍历数据
1 | students1.ForEach(stu => Console.WriteLine(stu)); |
Select 投影 将原始类(一般为po)的属性投影到新的类(一般为dto)的属性中
1 | var studentsWithIdAndName = studentList.Select(stu => new {Id=stu.Id,Name=stu.Name}).ToList(); |
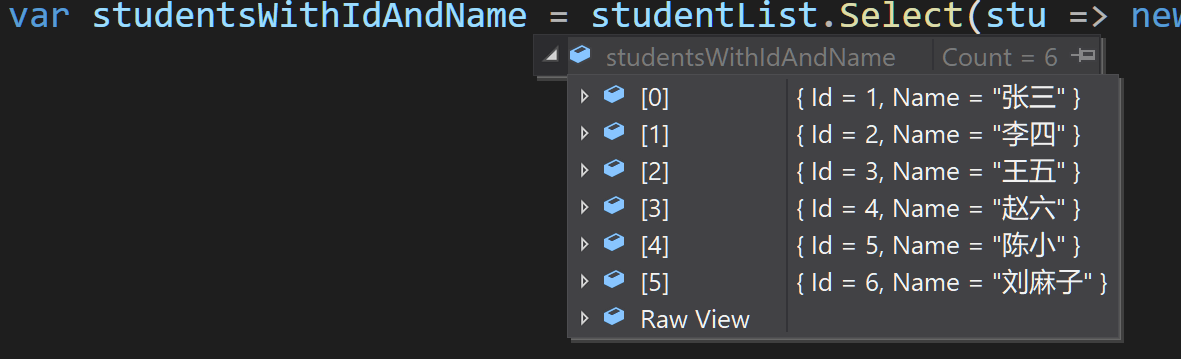
Join 内连接
1 | var studentsWithClass = studentList.Join(stuClasses,stu=>stu.ClassId,clazz =>clazz.Id, |
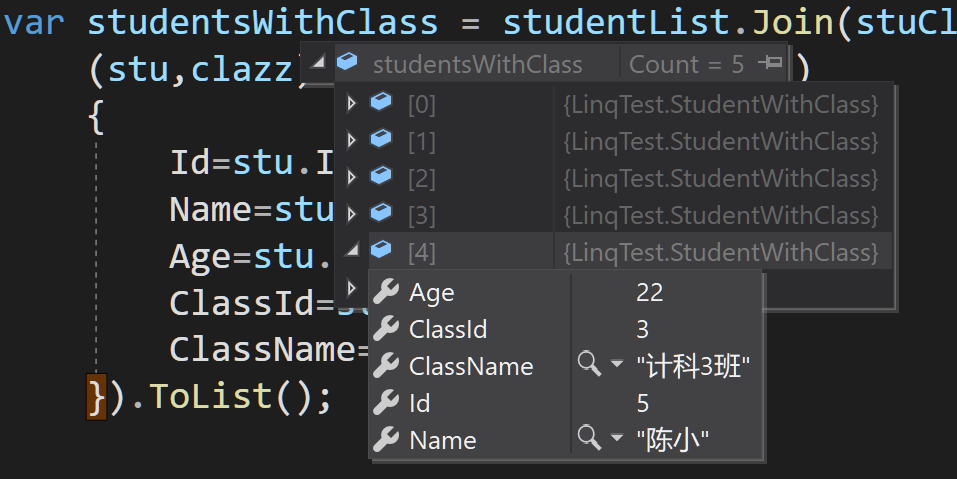
Take 获取指定数量的数据
Skip 跳过指定数量的数据
OrderBy 根据指定元素升序排列
OrderByDescending 根据指定元素降序排列
Where + Contains 模糊查询
1 | var students2 = studentList.Where(stu => stu.Name.Contains("张")).OrderByDescending(stu => stu.Age).ToList(); |
GroupBy 指定属性进行分组
1 | IEnumerable<IGrouping<int,Student>> items = studentList.GroupBy(s => s.Age); |